I was developing a feature in my app, in particular a map with some icon markers using this package. During my programming session I used an Icon widget and I set the icon size at 100. Good, everything run smoothly, but when I launched the app on different screens I found the following situation here below.
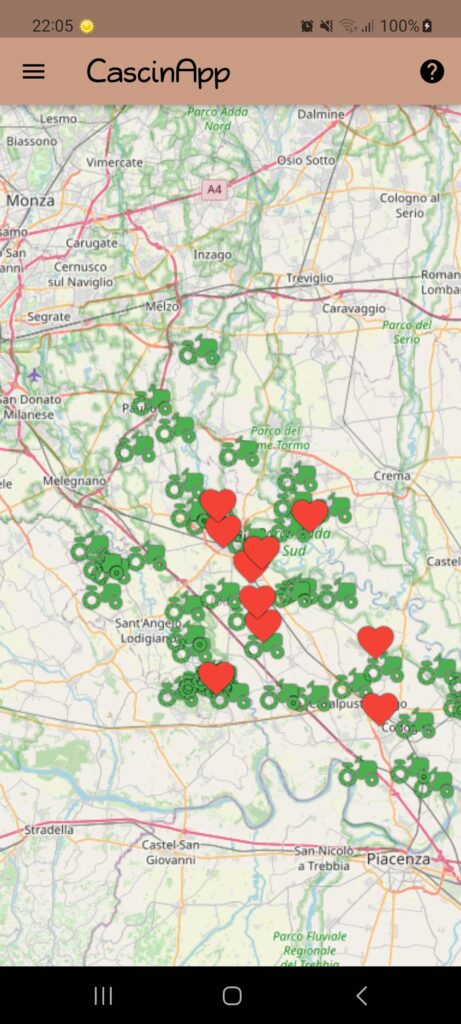
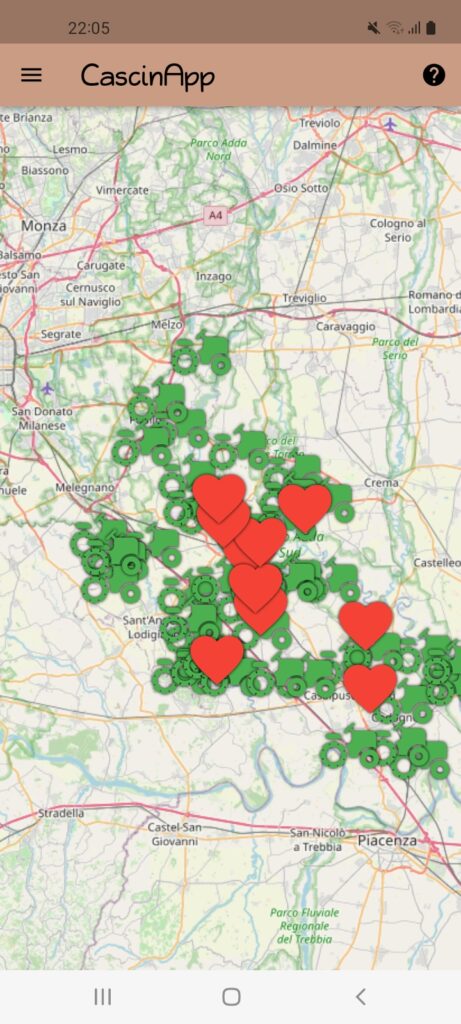
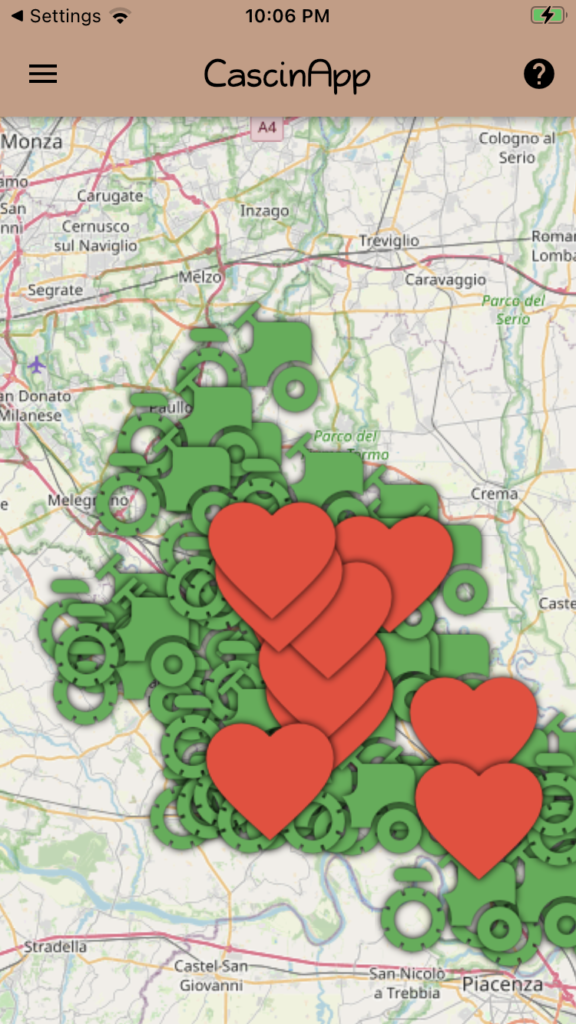
All the sizes were different! This depends on multiple factors such as the number of pixels and the Device Pixel Ratio. In fact, you have to consider that Flutter works on the concept of “logical pixel” that is independent across multiple devices.
To achieve my goal I had to find a way to have a constant size in all of the smartphones. I explain here the hack I used to solve this problem.
First of all I had to know how many logical pixels each device had. To do this I used the following instructions:
double width = MediaQuery.of(context).size.width;
double height = MediaQuery.of(context).size.height;
Then I had to know the DPR:
double ratio = MediaQuery.of(context).devicePixelRatio;
Everything I was looking for was retrieved. The next step was to multiply the dpr with the total numbers of logical pixel on the screens. Here a table of the approximated values:
Galaxy A52 | Galaxy A21 | iPhone 8 | |
Logical px (width * height) | 310.000 | 350.000 | 250.000 |
DPR | 2,81 | 1,75 | 2,0 |
Logical px * DPR = total number of physical px | 871.000 | 612.500 | 500.000 |
The next step was to find an adequate size for the icon in logical pixels for the smaller phone (the iPhone) and the bigger one (the Samsung Galaxy A21).
In my case I decided to use size = 100 for the Galaxy A52 and size = 40 for the iPhone8 to try to normalize the different icons sizes.
Once given the maximum and the minimum values there was just a thing to do: calculate the equation of the line from two points. Let’s see here the complete formula with the values decided previously:
double x1 = 871000;
double y1 = 100;
double x2 = 500000;
double y2 = 40;
double x = (width * height) * ratio;
double iconSize = (((x - x1) / (x2 - x1)) * (y2 - y1)) + y1;
Then I assigned iconSize to the size of the Icon in this way:
Icon(
Icons.agriculture,
color: Colors.green,
size: iconSize,
)
and this was the result:
Mission complete! 👍
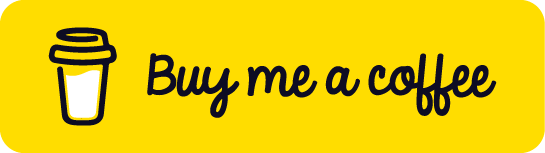
