Sometimes you may need to call different API in order to build a widget and you need all of these information at the same time. In Flutter it’s pretty easy to do this using the FutureBuilder widget and the wait() method.
Let’s see here an example:
import 'dart:convert'; import 'package:http/http.dart'; Future<dynamic> firstFuture() async { const String myEndpoint = "https://...."; Response response = await Client().get(Uri.parse(myEndpoint)); return jsonDecode(response.body)["data"]; } Future<dynamic> secondFuture() async { const String myEndpoint = "https://...."; Response response = await Client().get(Uri.parse(myEndpoint)); return jsonDecode(response.body)["data"]; }
Here above we have two futures calling two different endpoints. In these cases we are returning two json.
Let’s now have a look on how to use these methods during the build of our MaterialApp. Consider the following code:
FutureBuilder( future: Future.wait([firstFuture(), secondFuture()]), builder: (BuildContext context, AsyncSnapshot<dynamic> snapshot) { print(snapshot.hasData); if (snapshot.connectionState == ConnectionState.done && snapshot.hasData) { print("First future result: ${snapshot.data[0]}"); print("Second future result: ${snapshot.data[1]}"); return const MyWidget(); } else { return CircularProgressIndicator(); } }, ),
The FutureBuilder has a parameter called “future” where we can pass our list of futures using the Future.wait() method. In this way we can easily wait for futures, access then to both of the results and render more widgets.
Enjoy!
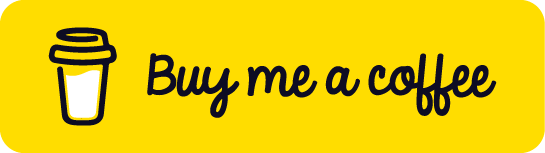
